Async operations
Fast-track the process of building and executing requests in an asynchronous format.
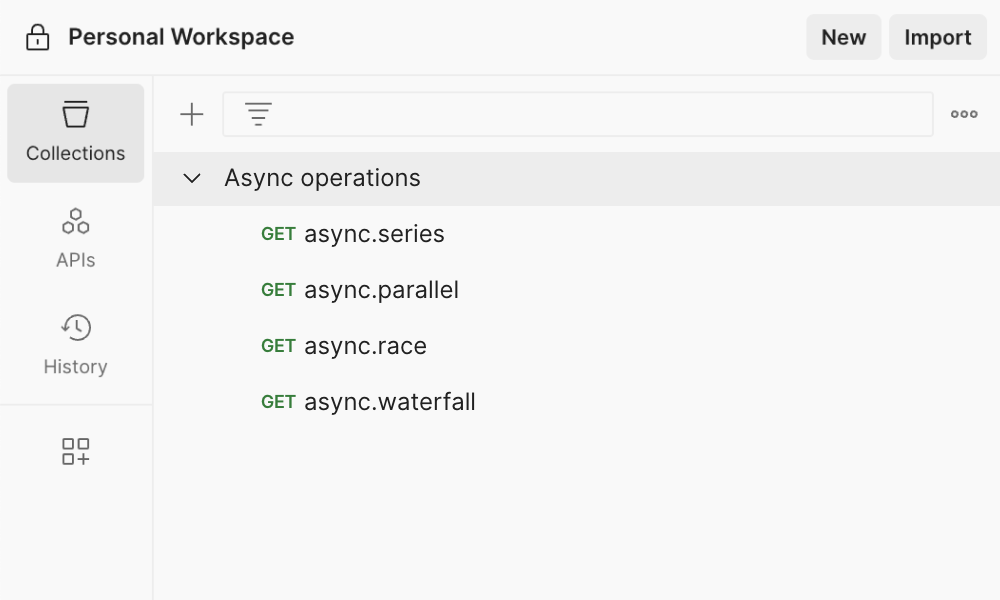
Overview
Asynchronous operations allow tasks to be executed independently, enabling efficient utilization of system resources and non-blocking execution. This template guides you through building and running requests in an async manner to ensure tasks are processed predictably and enhance your app’s performance.
What are asynchronous operations?
Asynchronous operations, or async operations, help you execute requests and handle responses independently of the main program or flow. You can use asynchronous operations to handle tasks like uploading multiple files to a server, web scraping, and updating shared resources. In the context of API development, asynchronous operations allow you to handle requests and responses concurrently without blocking the execution of other tasks. This asynchronous approach helps you enhance the performance and responsiveness of your application.
What does the asynchronous operations template contain?
The asynchronous operations template contains sample requests and functions that demonstrate how to set up and execute asynchronous operations in Postman. These examples showcase how to handle asynchronous tasks in your API workflow, allowing you to optimize the processing of requests and responses. The template includes the following functions -
async.series (sequential asynchronous execution)
async.parallel (concurrent asynchronous execution)
async.race (asynchronous race condition)
async.waterfall (chained asynchronous execution)
How to use the asynchronous operations template?
Follow these easy steps to use the asynchronous operations template effectively: Step 1. Explore the sample requests and functions: Go through the examples and understand how the functions are utilized to perform asynchronous operations. Step 2. Customize the template: Modify the sample requests and functions to match your API endpoints and desired asynchronous patterns. Update the request configurations, URLs, headers, and function logic as needed. Step 3. Execute the asynchronous operations: Send the requests and observe how the asynchronous operations are handled. Analyze the results, especially the order and timing of execution. Step 4. Incorporate into your workflow: Based on your requirements, implement relevant asynchronous patterns given in the template. Integrate them into your existing workflows or use them as a reference to set up asynchronous operations in your API development.
Get started fast. Fork and customize this template in Postman
Use TemplateFrequently asked questions
Who can use the template?
The asynchronous operations template is suitable for various roles involved in API development, including Backend Developers, Fullstack Developers, Frontend Developers, and Quality Engineers. Anyone seeking to optimize the performance and responsiveness of their API applications can benefit from using this template.
What are some applications of asynchronous operations?
Here are a few common applications of asynchronous operations:
File input/output: Reading from or writing to files asynchronously.
Network operations: Performing network requests, such as HTTP requests or socket communication asynchronously.
Database operations: Executing database queries or transactions asynchronously.
Timers and delays: Setting up timers or scheduling tasks to be executed after a specific delay or at a specific time.
Event handling: Processing user interactions, system events, or message passing between components asynchronously.
Parallel processing: Executing computationally intensive tasks in parallel by utilizing multiple CPU cores or distributed systems.
Task queues: Managing and processing tasks asynchronously from a queue, ensuring efficient utilization of resources and workload distribution.
Asynchronous messaging: Sending or receiving messages between components or systems to enable asynchronous communication and decoupling.
Asynchronous streaming: Processing continuous streams of data asynchronously, such as real-time data feeds or audio/video streaming.
Asynchronous task completion: Waiting for one or more tasks to complete and handling the results asynchronously.
Popular Templates
Integration testing
Verify how different API endpoints, modules, and services interact with each other.